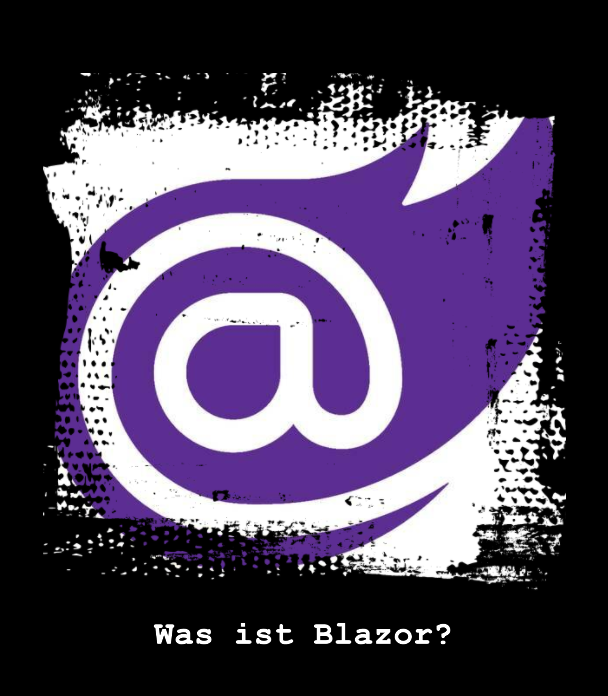
Let’s try to answer the question “What is Blazor” very briefly:
Blazor is an innovative web development framework from Microsoft that allows developers to create interactive web applications using C# instead of JavaScript. By using WebAssembly, a binary code technology, Blazor applications can run directly in the browser, resulting in a faster and smoother user experience. In this article, we will take an in-depth look at Blazor, exploring its history, architecture and basic concepts, as well as a look at the future of Blazor and its advantages over other web development technologies.
Contact
The story of Blazor: the birth of a new web development tool
Blazor was originally introduced by Microsoft developer Steve Sanderson as an experimental project in 2017. The name “Blazor” is a combination of “browser” and “Razor”, with Razor being a syntax developed by Microsoft for C# and HTML templates. Since its initial launch, Blazor has gone through several stages of development and was finally launched as an official Microsoft product in September 2019 with the release of .NET Core 3.0.
The success of Blazor is due to a combination of factors, including the growing popularity of WebAssembly, the strong ecosystem of the .NET Platform, and Microsoft’s commitment to providing innovative web development tools. Blazor has over time attracted a growing community of developers and companies who use the framework to build a variety of web applications, from simple interactive pages to complex business applications.
Steve Sanderson is rightly considered the father of Blazor. His ability to design great technology and then present it in a humble and concise way makes him a pleasure to listen to. If you have some time, you might want to watch this presentation video at the NDC conference to see his answer to the question “What is Blazor”:
Basic concepts and architecture of Blazor
Blazor is based on a component-oriented approach in which web applications are created from reusable and independent components. Each Blazor component consists of HTML, CSS and C# code that is responsible for rendering and interacting with the user interface. The components communicate with each other via parameters, events and cascading values to exchange data and information.
Blazor’s architecture follows the Model-View-Update (MVU) pattern, which supports a clear separation of responsibilities and unidirectional data flow control. In Blazor, the developer updates the application state by responding to events triggered by user interactions. The user interface is then automatically updated to reflect the new state.
Blazor hosting models: Server and WebAssembly
Blazor offers two hosting models for web applications: Blazor Server and Blazor WebAssembly. Both models have their own advantages and disadvantages and are suitable for different use cases.
Blazor Server applications run on a server and use SignalR, a real-time communication library, to exchange user interactions and UI updates between the server and the browser. Blazor Server offers a fast start-up time because the application is pre-rendered on the server and only the necessary UI updates are sent to the browser. However, this architecture can lead to a reduced user experience when network latency is high and requires a constant internet connection.
Blazor WebAssembly, on the other hand, is client-side and runs directly in the user’s browser. The application is downloaded and executed as WebAssembly code, eliminating the need for a constant server connection. Blazor WebAssembly offers better scalability and reduces server load as all processing takes place on the client. However, application start-up times are typically longer because all code and resources must be downloaded.
Comparison of the models
The following table shows the main differences between the two hosting models:
Features | Blazor Server | Blazor WebAssembly |
---|---|---|
Hosting | Server-side | Client-side (in browser) |
Communication | SignalR (Real-time- Communication) | Direct browser interaction |
Internet connection | Permanent connection required | No permanent connection required |
Start-up time | Faster, as pre-rendered | Longer, as all code is downloaded |
Scalability | Limited by server resources | Better scalability, client-side processing |
Server load | Higher, as UI updates are managed by the server | Lower, as processing is done in the browser |
Network latency | May result in reduced user experience | Less affected |
Off-line Capability | Not supported | Supported |
Browser API integration | Indirect via SignalR | Direct integration |
Debugging | Easier, since server-side | More complex, since client-side |
Sometimes a hybrid approach can be useful, where the Blazor app is developed in such a way that you can quickly switch between the two models. You have to choose one model when publishing the app, but you can switch back and forth during development. This way, you can combine the advantages of fast start-up time and easy debugging at development time with the scalability advantages of WebAssembly.
Blazor components: The building blocks of a Blazor application
Blazor components are the basic building blocks of any Blazor application. They consist of C# code and Razor markup and allow the creation of reusable UI elements that can easily be used in other components or applications. A Blazor component can contain parameters that allow it to get data and settings from parent components, and can also trigger its own events to send notifications of internal changes to parent components.
In Blazor, developers can create both simple and complex components that manage both static and dynamic content. Examples of components include buttons, input fields, dropdown menus, dialogue boxes and even complete layouts that form the framework for an application.
Out of the box, Blazor only comes with a very small number of components. However, it is very easy to extend html tags with C# code so that the desired result appears on the screen. For example, a dynamic html list can be created with code like this:
Here you can see how easily C# code can be used to dynamically generate html directly in the .razor file. Of course, you enjoy features that are made possible by the static types of C#, such as syntax highlighting and auto-completion.
If you want to reuse the code, it is very easy to create a component from Razor code, C# code and, if necessary, CSS and JavaScript. It is up to the developer whether he wants to put everything in one file or whether he benefits from the possibility to write everything or parts in separate files. Most IDEs group these files automatically so that the overview is not lost.
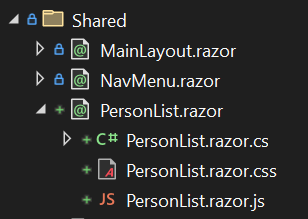
Here you can see how the PersonList component was split into four files: A .razor file containing the mix of Html and C# for the display, a .CS file containing pure C# code (e.g. for event handling or asynchronous data loading), a CSS file for styles and a .js file with JavaScript.
Interaction with JavaScript in Blazor applications
Although Blazor is primarily based on C#, JavaScript interaction is still possible in Blazor applications and is often necessary to access certain browser APIs or to use existing JavaScript libraries. Blazor provides JavaScript Interop, a feature that allows JavaScript functions to be called from C# code and vice versa.
To include JavaScript in Blazor, developers can use the IJSRuntime interface, which provides methods for calling JavaScript functions and receiving return values. When doing so, it is important to ensure that communication between C# and JavaScript is asynchronous to ensure a smooth user experience.
JS interop calls are fundamentally designed to be asynchronous, regardless of whether the called code itself is synchronous or asynchronous. The asynchronous default design ensures that components are compatible with both Blazor hosting models – Blazor Server and Blazor WebAssembly. In the case of Blazor Server, it is necessary for all JS interop calls to be asynchronous, as they are transmitted over a network connection. In contrast, synchronous JS interop calls are also possible for applications that rely exclusively on the Blazor WebAssembly hosting model.
An Example
There are several ways to combine JavaScript and Blazor. If you have JavaScript code that was written exactly for one component, it is recommended to write the JavaScript code in a file with the same name as the Blazor component + file extension ‘.js’. This way the code is automatically bundled and can be easily referenced in the component.
In my example, I wrote some JavaScript in the file PersonList.razor.js:
To use the code from C# (file PersonList.razor.cs), overwrite the method OnAfterRenderAsync() and import the JavaScript code. Afterwards, the JavaScript functions are available for a call in C#:
Data binding and event handling in Blazor
Data binding is a central concept in Blazor that allows data to be seamlessly synchronised between UI elements and C# code. Blazor supports both one-way and two-way data binding, allowing developers to customise data binding according to their needs. One-way data binding binds the data source to a UI element so that changes to the data source are automatically reflected in the UI element. Two-way data binding, on the other hand, allows changes in the UI element to be automatically updated in the data source as well.
Event handling is another important aspect of Blazor that allows developers to respond to user interactions and other events within the application. In Blazor applications, developers can add event handlers for various events such as clicks, inputs or hover, which then call C# methods to perform corresponding actions.
An Example
In this example, we create a simple Blazor component that demonstrates two-way data binding. The component contains an input field and a text that displays the content of the input field in real time.
For example, create a new Razor component in the TwoWayBinding.razor file with this content:
In this example, we bind the value of the input field to the InputText property with the @bind directive. This achieves two-way data binding: changes in the input field are automatically updated in the InputText property, and vice versa.
Let’s now add a link to the component in the NavMenu.razor file so that the component is accessible in the application:
Routing and navigation in Blazor applications
Blazor provides a built-in routing system that allows you to create simple and user-friendly URLs for different pages and components within the application. The routing system is based on the @page directive used in Razor files to specify the route path of a component or page.
The Blazor routing system also supports parameters and optional parameters that allow additional information to be passed to components and provide flexibility to navigation within the application. Developers can also access the NavigationManager object to enable programmatic navigation within the application.
An Example
Let’s create a new Razor component (e.g. MyParameterizedComponent.razor) with the following content:
In this example, we use the @page directive to set the route for the component. The route contains placeholders for two parameters: RequiredParam and OptionalParam. The RequiredParam is a mandatory parameter, while the OptionalParam is of type int? and is optional.
Blazor Extensions and Community Resources: The Blazor Community Evolves
The growing Blazor community has helped to provide a variety of extensions, libraries and tools for Blazor application development. These resources make it easier for developers to quickly and efficiently implement features and solutions in their applications.
Popular Blazor extensions include UI libraries such as Radzen Blazor Components, DevExpress Blazor and Syncfusion Blazor, which provide a large number of pre-built components and controls for creating engaging user interfaces. In addition, there are also many resources such as blogs, tutorials, videos and online courses to help developers deepen their Blazor knowledge and expand their skills.
In addition to the commercial components, there are now also a number of really good libraries from the open source sector. For me personally, MudBlazor stands out here. You notice that the library fits together and can be used directly with little code. In addition, MudBlazor offers an appealing design and a really extensive collection of components. A small shortcoming of MudBlazor is the lack of native support for OData, at least for the time being.
Comparing component libraries
The following table compares the key features of four Blazor component libraries: Blazor Radzen, DevExpress Blazor, Syncfusion Blazor and MudBlazor. The table includes information about the licence, number of components, design style, responsiveness, mobile support, supported browsers, documentation, community, template customisation and support. Note that this information may vary depending on updates and changes to the respective libraries.
Merkmale | Blazor Radzen | DevExpress Blazor | Syncfusion Blazor | MudBlazor |
---|---|---|---|---|
License | Proprietär (Freemium) | Proprietär (Freemium) | Proprietär (Freemium) | Open Source (MIT) |
Numer of components | More than 60 | More than 65 | More than 65 | More than50 |
Design- Stil | Radzen Theme | DevExpress Themes | Syncfusion Themes | Material Design |
Responsive | Yes | Yes | Yes | Yes |
Mobile Support | Yes | Yes | Yes | Yes |
Supported Browsers | Modern Browsers | Modern Browsers | Modern Browsers | Modern Browsers |
Documentation | Extensive | Extensive | Extensive | Extensive |
Community | Growing | Growing | Growing | Growing and very active |
Template- Adoption | Yes | Yes | Yes | Yes |
Support | Free (Community) Paid (Enterprise) | Free (Community) Paid (Enterprise) | Free (Community) Paid (Enterprise) | Free (Community) |
Future perspectives and advantages of Blazor compared to other web development technologies
Blazor has established itself as a promising alternative to traditional web development technologies such as Angular, React and Vue.js. By using C# and .NET, Blazor allows developers to access a unified ecosystem for all application development, which increases productivity and maintainability.
Another advantage of Blazor is its support for WebAssembly, which offers better performance and speed for web applications. As WebAssembly continues to grow in popularity and browser manufacturers
Conclusion: What is Blazor and what can it be used for?
Blazor is an innovative and powerful web application development framework based on .NET and C#. It enables developers to create client-side web applications with a familiar programming language and a unified code base for backend and frontend. Blazor is particularly well suited for business-specific applications that focus on interaction with data and business logic.
Although Blazor is being continuously developed and offers many advantages, it still has some catching up to do with public websites compared to other frameworks such as React or Angular. Here, aspects such as performance and SEO can be crucial, and there are areas where Blazor is not yet as mature as the established alternatives.
It is important to emphasise that no tool or framework is equally well suited to all use cases. Careful consideration of specific requirements and objectives should always be made when choosing the right technology for a project. Nevertheless, Blazor offers a compelling option for many scenarios, especially for developers who are already familiar with .NET and C#.
Personally, I try to use Blazor in all projects that are suitable for it because it is really efficient and scalable.
Pingback: Exploring the MudThemeProvider in MudBlazor: A Comprehensive Guide - crispycode.net