Welcome to the ultimate Blazor Cheat Sheet, a comprehensive resource designed to accelerate your Blazor development journey. Whether you’re a seasoned developer or a beginner stepping into the world of Blazor, this cheat sheet is your go-to guide for quick, easy-to-understand snippets and examples.
This cheat sheet covers a wide range of topics, from setting up Blazor and creating components, to advanced topics like authentication, authorization, and deployment. Each snippet in the cheat sheet is linked to a more detailed example and explanation, providing you with a deeper understanding of how to implement and use these features in your own Blazor applications.
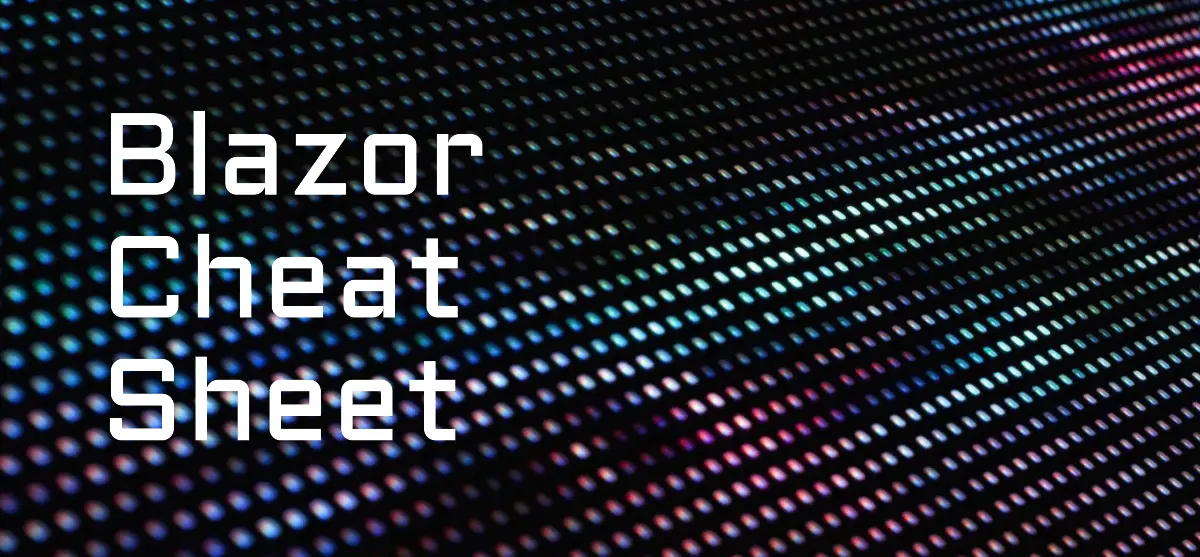
Table of Contents
Routing and Navigation Blazor Cheat Sheet
Basic routing setup in a Blazor application
To set up basic routing in a Blazor application, you would follow these steps:
Define the Route: In your Blazor component, you can define a route using the @page
directive at the top of the file. For example, if you have a component named Index
, you can define the route as @page "/index"
.
Link to the Route: You can use the NavLink
component to create links to your routes. The NavLink
component generates an <a>
element and automatically adds the active
class if the current URL matches the href
attribute.
Set Up the Router: In your App.razor
file, set up the Router
component. The Router
component uses a RouteTable
to render the component that corresponds to the current URL.
In this example, MainLayout
is the default layout for the application, and the NotFound
section defines what to display when no matching route is found.
Navigate Programmatically: You can also navigate programmatically using the NavigationManager
service. You can inject it into your component and use the NavigateTo
method.
Remember to always ensure that the route you’re navigating to has been defined using the @page
directive in the corresponding component.
Dynamic route handling based on user input or actions in Blazor
To handle dynamic routing based on user input or actions in a Blazor application, you can use route parameters and the NavigationManager
service. Here’s how you can do it:
Define the Route with Parameters: In your Blazor component, you can define a route with parameters using the @page
directive. The parameters are defined in the route template as segments enclosed in braces {}
.
In this example, id
is a route parameter. When you navigate to /product/5
, the Id
property in the component gets the value 5
.
Link to the Route with Parameters: You can use the NavLink
component to create links to your routes with parameters.
Navigate Programmatically with Parameters: You can also navigate programmatically using the NavigationManager
service. You can inject it into your component and use the NavigateTo
method.
In this example, when the button is clicked, the application navigates to the product page for product 5.
Handle route constraints
Route constraints in Blazor allow you to restrict the values that can be matched by a route parameter. They are specified in the route template by appending a colon :
and the constraint name after the parameter name. Here’s how you can use them:
Define the Route with Constraints: In your Blazor component, you can define a route with constraints using the @page
directive. The constraints are defined in the route template as segments enclosed in braces {}
.
In this example, id
is a route parameter with an int
constraint. This means that the route will only match if the id
segment of the URL is an integer. If you navigate to /product/5
, the Id
property in the component gets the value 5
. If you navigate to /product/abc
, the route does not match and the component is not rendered.
Use Multiple Constraints: You can use multiple constraints for a single parameter by separating them with a colon :
.
In this example, the id
parameter has two constraints: int
and min(1)
. This means that the route will only match if the id
segment of the URL is an integer greater than or equal to 1.
Blazor supports several built-in constraints, including bool
, datetime
, decimal
, double
, float
, guid
, int
, long
, and min(value)
. You can also create custom constraints by implementing the RouteConstraint
interface.
Use NavigationManager to get the current URL or to navigate to a new URL
The NavigationManager
service in Blazor provides functionalities for working with URIs and navigation events. Here’s how you can use it:
Inject the NavigationManager Service: First, you need to inject the NavigationManager
service into your component using the @inject
directive.
Get the Current URI: You can use the Uri
property of the NavigationManager
service to get the current URI.
In this example, the OnInitialized
method gets the current URI when the component is initialized.
Navigate to a New URI: You can use the NavigateTo
method of the NavigationManager
service to navigate to a new URI.
In this example, when the button is clicked, the application navigates to the /product
route.
Listen for Navigation Events: You can use the LocationChanged
event of the NavigationManager
service to listen for navigation events.
In this example, the HandleLocationChanged
method is called every time the location changes.
Handle 404 errors or redirect to a custom error page in Blazor
Handling 404 errors or redirecting to a custom error page in a Blazor application can be done using the Router
component in the App.razor
file. Here’s how you can do it:
Create a Custom Error Component: First, create a Blazor component that will serve as your custom 404 error page. This component can contain any content you want to display when a user tries to navigate to a route that doesn’t exist.
Set Up the Router: In your App.razor
file, set up the Router
component to use your custom error component. The Router
component uses a RouteTable
to render the component that corresponds to the current URL. If no matching route is found, it renders the component specified in the NotFound
section.
In this example, MainLayout
is the default layout for the application, and the NotFound
section defines what to display when no matching route is found.
Redirect to the Custom Error Page: If you want to redirect to the custom error page instead of displaying the error message in place, you can use the NavigationManager
service to navigate to the error page.
In this example, when no matching route is found, the application navigates to the /notfound
route, which displays the custom error page.
Programmatically navigate between pages in Blazor
To programmatically navigate between pages in a Blazor application, you can use the NavigationManager
service. Here’s how you can do it:
Inject the NavigationManager Service: First, you need to inject the NavigationManager
service into your component using the @inject
directive.
Navigate to a Route: You can use the NavigateTo
method of the NavigationManager
service to navigate to a specific route. This method takes a string parameter which is the route you want to navigate to.
In this example, when the button is clicked, the application navigates to the /product
route.
Navigate with Parameters: If you want to navigate to a route with parameters, you can include the parameters in the route string.
In this example, when the button is clicked, the application navigates to the product page for product 5.
Remember to always ensure that the route you’re navigating to has been defined using the @page
directive in the corresponding component.
Secure routes and implement authorization in Blazor
Securing routes and implementing authorization in a Blazor application involves a few steps. Here’s how you can do it:
- Install the Necessary Packages: First, you need to install the necessary NuGet packages for authentication and authorization. These packages include
Microsoft.AspNetCore.Components.Authorization
andMicrosoft.AspNetCore.Authorization
. - Set Up the Authentication State Provider: In your
Startup.cs
file, you need to set up the authentication state provider. This provider is responsible for determining the authentication state of the user.
RevalidatingIdentityAuthenticationStateProvider
is a built-in class in ASP.NET Core that provides an AuthenticationStateProvider
implementation. This class is designed to work with ASP.NET Core Identity and it revalidates the user’s authentication state at regular intervals.
AuthenticationStateProvider
is a service that provides information about the authentication state of the current user. It has a method called GetAuthenticationStateAsync
which returns an AuthenticationState
object representing the current user. This object includes a ClaimsPrincipal
representing the user. If the user is not authenticated, the ClaimsPrincipal
will be an anonymous user.
In a Blazor application, you can use the AuthenticationStateProvider
to get the current user’s authentication state and display different content based on whether the user is authenticated or not.
The RevalidatingIdentityAuthenticationStateProvider
extends the AuthenticationStateProvider
and adds revalidation functionality. This means that it periodically checks whether the user’s authentication state is still valid. If the user’s authentication state is no longer valid (for example, if their login session has expired), the RevalidatingIdentityAuthenticationStateProvider
will update the authentication state to reflect that the user is no longer authenticated.
This class is typically used in Blazor Server applications where the user’s authentication state is stored in the server’s memory and needs to be revalidated at regular intervals. In a Blazor WebAssembly application, you would typically use the AuthenticationStateProvider
provided by the Microsoft.AspNetCore.Components.WebAssembly.Authentication
package.
Protect Your Routes: In your Blazor components, you can use the Authorize
attribute to protect your routes. This attribute ensures that only authenticated users can access the route.
Use the AuthorizeView Component: You can use the AuthorizeView
component to display different content based on the authentication state of the user.
Implement Role-Based Authorization: If you want to implement role-based authorization, you can use the Authorize
attribute with the Roles
parameter.
In this example, only users with the “Admin” role can access the /admin
route.
Remember that you need to set up an authentication mechanism (like Identity or JWT authentication) to authenticate your users before you can use these authorization features.
Handle query strings in Blazor routing
Handling query strings in Blazor routing involves parsing the query string parameters from the URL. Here’s how you can do it:
Inject the NavigationManager Service: First, you need to inject the NavigationManager
service into your component using the @inject
directive.
Get the Query String Parameters: You can use the Uri
class and the NavigationManager
service to get the query string parameters. The Uri
class can parse the URL and provide access to the query string parameters.
In this example, when the component is initialized, it gets the id
parameter from the query string.
Navigate with Query String Parameters: If you want to navigate to a route with query string parameters, you can include the parameters in the route string.
In this example, when the button is clicked, the application navigates to the product page with the id
parameter in the query string.
Remember that query string parameters are not the same as route parameters. Route parameters are part of the route template, while query string parameters are appended to the URL after a ?
character.
Force a re-render of a component when parameters change
To force a re-render of a component when parameters change in Blazor, you can use the OnParametersSet
or OnParametersSetAsync
lifecycle methods. These methods are called every time a parameter is set, even if its value hasn’t changed. Here’s how you can do it:
In this example, the OnParametersSet
method is overridden to run custom logic every time the Id
parameter is set. This forces the component to re-render, even if the Id
hasn’t changed.
Remember that the OnParametersSet
and OnParametersSetAsync
methods are called after parameter values are set but before the component is rendered. This makes them the perfect place to put logic that needs to run when parameters change.
Other ways to force a re-render of a component in Blazor
Change the State: The most common way to force a re-render of a component in Blazor is to change the state of the component. This can be done by changing the value of a property or field in the component. When the state changes, Blazor automatically re-renders the component.
In this example, clicking the button increments the counter
variable, which causes the component to re-render.
Use the StateHasChanged Method: You can call the StateHasChanged
method to tell Blazor to re-render the component. This can be useful in scenarios where the state change is not detected automatically.
In this example, clicking the button calls the StateHasChanged
method, which forces the component to re-render.
Use Event Callbacks: If you have a parent and child component, you can use an EventCallback
to notify the parent component when the state of the child component changes. The parent component can then force a re-render of the child component.
In this example, the HandleChange
method is called when the OnChange
event is triggered in the ChildComponent
. The HandleChange
method then calls StateHasChanged
to force a re-render of the parent component.
Remember that forcing a re-render of a component can be expensive in terms of performance, so it should be done sparingly. It’s usually better to let Blazor handle the rendering based on its built-in change detection.
Handle prerendering in Blazor
Prerendering in Blazor Server is a feature that allows the application to render the initial HTML of a component on the server before it’s sent to the client. This can improve the perceived load time of the application, as the user can see the initial state of the application before any JavaScript is run.
Here’s how you can enable prerendering in a Blazor Server application:
Update the Host Page: In your _Host.cshtml
file (or _Host.razor
in .NET 6 and later), you can use the component
tag helper to render a Blazor component. To enable prerendering, set the render-mode
attribute to ServerPrerendered
.
In this example, the Main
component is prerendered on the server.
Handle State: When a component is prerendered, it’s rendered twice: once on the server and once on the client. If your component has any state that needs to persist across these two renders, you need to handle it manually. You can do this by injecting a service into your component and storing the state in the service.
Use routing in Blazor to create a multi-language application
Creating a multi-language application in Blazor with routing involves a few steps. Here’s how you can do it:
Define the Route with Language Parameter: In your Blazor component, you can define a route with a language parameter using the @page
directive. The language parameter is defined in the route template as a segment enclosed in braces {}
.
In this example, lang
is a route parameter. When you navigate to /en/product
, the Lang
property in the component gets the value en
.
Localize the Component: You can use the IStringLocalizer
service to localize the text in your component based on the Lang
parameter.
In this example, the IStringLocalizer<Product>
service is injected into the component and used to localize the text. The localized text is retrieved using the indexer of the Localizer
object.
Set the Culture: You can use the CultureInfo
class to set the culture of the current thread based on the Lang
parameter. This will affect the formatting of dates, numbers, and other culture-specific values.
In this example, the OnParametersSet
method sets the culture of the current thread when the Lang
parameter is set.
Remember that you need to provide the localized text for each language you want to support. This is typically done using .resx files or a similar resource file format. You also need to handle the case where the Lang
parameter is not a valid language code.
Component Communication Blazor Cheat Sheet
Passing parameters to a component
Passing parameters to a Blazor component is a common practice when you want to pass data from a parent component to a child component. Here’s how you can do it:
Define the Parameter in the Child Component: In your child component, you can define a parameter using the [Parameter]
attribute. This attribute tells Blazor that the property can be used as a parameter.
In this example, Title
is a parameter that can be set by the parent component.
Pass the Parameter from the Parent Component: In your parent component, you can pass a value to the child component’s parameter using attribute syntax.
In this example, the Title
parameter of the ChildComponent
is set to “Hello, World!”.
Pass Multiple Parameters: You can pass multiple parameters to a child component by defining multiple properties with the [Parameter]
attribute.
In this example, the Title
and Subtitle
parameters of the ChildComponent
are set.
Remember that parameters are set by the parent component and should not be modified by the child component. If the child component needs to modify the value, it should use an EventCallback
to notify the parent component of the change.
Use EventCallback to handle events in parent components
EventCallback is a delegate type in Blazor that’s used for component event handling. It’s designed to work with UI events that are triggered by user interactions like button clicks, text input, etc.
Here’s how you can use EventCallback to handle events in parent components:
Define the EventCallback in the Child Component: In your child component, you can define an EventCallback using the EventCallback
or EventCallback<T>
type.
In this example, OnClick
is an EventCallback that’s triggered when the button is clicked.
Handle the Event in the Parent Component: In your parent component, you can handle the EventCallback using attribute syntax. The method you specify will be called when the EventCallback is triggered.
In this example, the HandleClick
method is called when the OnClick
EventCallback in the ChildComponent
is triggered.
Pass Data with the EventCallback: If you want to pass data from the child component to the parent component, you can use the EventCallback<T>
type.
In this example, the OnClick
EventCallback passes the string “Hello, World!” to the parent component.
Remember that EventCallbacks should be invoked using the InvokeAsync
method. This ensures that the UI is updated correctly after the event is handled.
Cascading Parameters and Cascading Values
Cascading parameters and cascading values are a feature in Blazor that allows you to pass values from a parent component to its descendants without having to pass them through all the intermediate components. Here’s how you can use them:
Define the Cascading Value: In your parent component, you can define a cascading value using the CascadingValue
component. The Value
parameter specifies the value to cascade down to the child components.
In this example, the string “Hello, World!” is cascaded down to the ChildComponent
.
Receive the Cascading Parameter: In your child component, you can receive the cascading value using the [CascadingParameter]
attribute.
In this example, the Message
property receives the cascading value from the parent component.
Use a Name for the Cascading Value: If you have multiple cascading values, you can use the Name
parameter to specify a name for each cascading value.
In this example, the cascading value is named “Message”. The child component can use this name to receive the correct cascading value.
Note: If a name was provided, you must use the name in the CascadingParameter as well. Otherwise the value will always be null:
Remember that cascading values are a way to pass values down the component tree. They should not be used as a replacement for proper state management or communication between unrelated components.
Shared service for state management and communication
Using a shared service for state management and communication in Blazor is a common practice, especially in larger applications. Here’s how you can do it:
Create the Service: First, create a service that will hold the shared state and any methods needed to manipulate that state.
In this example, the StateService
holds a counter state and a method to increment the counter.
Register the Service: In your Startup.cs
file (or Program.cs
in .NET 6 and later), register the service with the dependency injection container.
In this example, the StateService
is registered as a singleton, which means that the same instance will be used throughout the application.
Inject the Service into Your Components: In your Blazor components, you can inject the service using the @inject
directive.
In this example, the StateService
is injected into the component and used to display and increment the counter.
Remember that services registered as singletons or scoped services will hold their state for the lifetime of the application or connection, respectively. This can be useful for sharing state, but you should be careful to manage this state appropriately to avoid unexpected behavior.
Use the @ref directive to create a reference to a child component
The @ref
directive in Blazor creates a reference to a child component. This reference can be used to invoke methods on the child component or access its properties. Here’s how you can do it:
Define the Child Component: In your child component, you can define a method or property that you want to access from the parent component.
In this example, the ChangeTitle
method changes the Title
property of the component.
Create a Reference to the Child Component: In your parent component, you can create a reference to the child component using the @ref
directive.
In this example, the childComponent
field holds a reference to the ChildComponent
. The ChangeChildTitle
method uses this reference to call the ChangeTitle
method on the child component.
Remember that the @ref
directive should only be used when necessary. In most cases, parent-child communication should be done using parameters and EventCallbacks. Using @ref
to manipulate child components can lead to hard-to-debug issues and tightly coupled code.